9.5. Eigenvalues and Eigenvectors#
import numpy as np
from numpy import linalg
9.5.1. Eigenvalues and Eigenvectors#
This section introduces eigenvalues and eigenvectors of a square matrix and explores some of their applications. The goal of this section is to dissect the action of linear maps into elements that are easy to visualize.
9.5.2. Motivation#
Consider a linear map
A = np.array([[3,-2], [1,0]])
u = np.array([2,1])
A @ u
array([4, 2])
We can see that the image of
In fact,
import matplotlib.pyplot as plt
def plot_map(vector, matrix):
x1 = vector[0]
y1 = vector[1]
x2 = (A @ vector)[0]
y2 = (A @ vector)[1]
ax = plt.axes()
ax.arrow(0, 0, x1, y1, head_width = 0.2,head_length = 0.2, fc ='b', ec ='b')
ax.arrow(0, 0, x2, y2, head_width = 0.2,head_length = 0.2, fc ='r', ec ='r')
ax.text(x1,y1 - 0.5,'$X$')
ax.text(x2,y2-0.5,'$AX$')
z = max(np.abs(x1), np.abs(x2), np.abs(y1),np.abs(y2))
ax.set_xticks(np.arange(-z-2, z+2, step = 1))
ax.set_yticks(np.arange(-z-2, z+2, step = 1))
ax.set_aspect('equal')
ax.set_xlabel("X")
ax.set_ylabel("Y")
plt.grid()
plt.show()
plot_map(u, A)
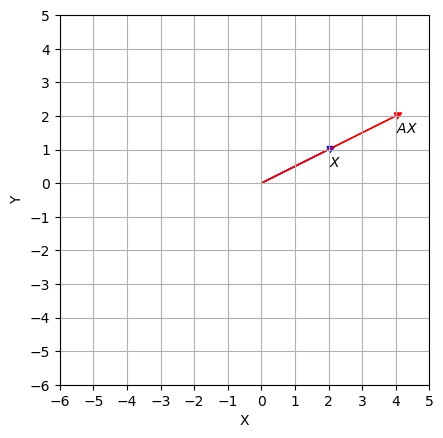
We are interested in such special vectors that when transformed by matrix
If
Example 1
Consider the matrix equation:
Observe that
a. Is
b. Is
c. Show that
Solution:
(a) Let’s compute
v = np.array([1, -1])
A @ v
array([5, 1])
If there were a
Which is not possible for any value of
plot_map(v, A)
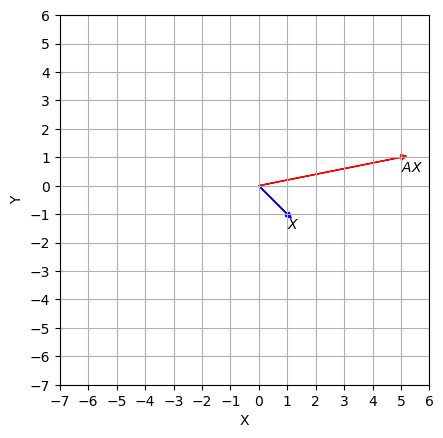
(b) No.
The homogeneous equation
# identity matrix I_2
I_2 = np.eye(2)
A - 4*I_2
array([[-1., -2.],
[ 1., -4.]])
Clearly, the columns of
(c)
A - I_2
array([[ 2., -2.],
[ 1., -1.]])
Since the columns of
Let’s set up the augmented matrix:
# the augmented vector
b = np.array([[0],[0]])
# add to the matrix
M = np.concatenate((A - I_2, b), axis = 1)
M
array([[ 2., -2., 0.],
[ 1., -1., 0.]])
Let’s recall the row operations:
def swap(matrix, row1, row2):
copy_matrix=np.copy(matrix).astype('float64')
copy_matrix[row1,:] = matrix[row2,:]
copy_matrix[row2,:] = matrix[row1,:]
return copy_matrix
# Replacing a row by the sum of itself and a multiple of another
def replace(matrix, row1, row2, scalar):
copy_matrix=np.copy(matrix).astype('float64')
copy_matrix[row1] = matrix[row1]+ scalar * matrix[row2]
return copy_matrix
M1 = swap(M, 0, 1)
M1
array([[ 1., -1., 0.],
[ 2., -2., 0.]])
M2 = replace(M1, 1, 0, -2)
M2
array([[ 1., -1., 0.],
[ 0., 0., 0.]])
A general solution for
and the set of all solutions is
each non-zero element of this set is an eigenvector corresponding to
The above simple example leads to a general case:
Theorem 17
Let
is an eigenvalue of . has non-trivial solution.
9.5.3. Eigenspace and characteristic polynomial#
Example 2
The set
Which we found in Example 1, is in fact the eigenspace
The polynomial
For each
Example 3
Suppose
We will find all eigenvalues and their multiplicities of
Solution:
The characteristic polynomial is given by:
Expanding the determinant, we obtain the characteristic polynomial:
The zeros of this polynomial (the eigenvalues of
Example 4
Let
Solution:
The eigenspace corresponding to
Therefore,
The eigenspace corresponding to
Therefore,
The eigenspace corresponding to
Therefore,
Before we move on to the next topic, let us check our calculation using numpy.linalg.eig
from NumPy linear algebra:
A = np.array([[4, -1, 6], [0, 1, 6], [0, 0, 8]])
evalues , evectors = np.linalg.eig(A)
for i in range(evalues.shape[0]):
print(evalues[i], ' is an eigenvector with ', evectors[:,i], ' as the corresponding eigenvectors')
4.0 is an eigenvector with [1. 0. 0.] as the corresponding eigenvectors
1.0 is an eigenvector with [0.31622777 0.9486833 0. ] as the corresponding eigenvectors
8.0 is an eigenvector with [0.69853547 0.46569032 0.54330537] as the corresponding eigenvectors
Note that numpy.linalg.eig
returns the unit vector of eigenvalues, and that is why we see different eigen vectors. To check that let’s normalize the eigenvector
v = np.array([9,6,7])
#normalizing v
e_v = v/np.linalg.norm(v)
e_v
array([0.69853547, 0.46569032, 0.54330537])
Let’s have another look at Example 2. The eigenvalues are the entries on the main diagonal. This is always true for triangular matrices and can be proved by induction:
Theorem 3
Let
Numerical Notes
Example 3 provides a method for manually computing eigenvectors in simple cases. In practical situations, using matrix program and row reduction method to find an eigenspace (for a specified eigenvalue) may not be entirely reliable. Roundoff error can occasionally lead to a reduced echelon form with the wrong number of pivots. The best computer programs compute approximations for eigenvalues and eigenvectors simultaneously, to any desired degree of accuracy, for matrices that are not too large.
Some software such as Mathematica and Maple can use symbolic calculations to find the characteristic polynomial of a moderate-sized matrix. But there is no formula or finite algorithm to solve the characteristic equation of a general matrix
for . The best numerical methods for finding eigenvalues avoid the characteristic polynomial entirely. In fact, MATLAB finds the characteristic polynomial of a matrix by first computing the eigenvalues of and then expanding the product
Exercises#
Exercises
If
is an eigenvector of corresponding to , what is ?If A is an
matrix and is an eigenvalue of , show that is an eigenvalue of .If
is an invertible matrix with eigenvalue show that has eigenvalueLet
a. Find the eigenvalues of
b. For each eigenvalue in part (a) find the corresponding eigenspace.
c. Find the dimension of each eigenspace in part (b) by finding a basis for it. How this result is related to your answer to part (a)?
9.5.4. Diagonalization#
This section introduces diagonalization and characterizes diagonalizability. Diagonalization is a useful factorization of a matrix
Computing powers of a matrix#
Let’s consider the matrix
Using this decomposition, we have:
Since
This decomposition, known as diagonalization, enables us to compute
Unfortunately, this decomposition doesn’t exist for all matrices. The goal of this section is to characterize matrices that admit such a factorization.
Diagonalization#
An
It turns out an
Theorem 18
An
In fact, suppose
Finding eigenvectors in general is not an easy task, and is not clear how to check which
Theorem 19
The eigenvectors corresponding to distinct eigenvalues are linearly independent.
Combining Theorem 18 and Theorem 19, we obtain the following corollary:
Corollary 1
An
Example 1
Is
diagonalizable? If yes find a diagonalization for it.
Solution
To determine if
Step 1
we use linalg.eigvas to find the eigenvalues and eigenvectors of
A = np.array([[1,3,3],[-3,-5,3],[3,3,1]])
# find evalues and evectors
evalues, evectors = linalg.eig(A)
print("eigenvalues = ", evalues)
eigenvalues = [-5. -2. 4.]
Step 2: Constructing the diagonal matrix
lambda_1 = evalues[0]
lambda_2 = evalues[1]
lambda_3 = evalues[2]
D = np.array([[lambda_1, 0, 0], [0, lambda_2, 0], [0, 0, lambda_3]])
D
array([[-5., 0., 0.],
[ 0., -2., 0.],
[ 0., 0., 4.]])
Step 3 : Finding the matrix
whose th column is an eigenvector corresponding to for :
# set up the invirtible matrix P
P = evectors
Step 4: compute the inverse of matrix P
# compute the inverse of P
Q = linalg.inv(P)
Step 5 (sanity check): Now that we have
, , , lets check if
P @ D @ Q
array([[ 1., 3., 3.],
[-3., -5., 3.],
[ 3., 3., 1.]])
Example 2
Is
diagonalizable? If yes find a diagonalization for it.
Solution:
Step 1: Finding eigenvalues and eigenvectors of
:
A = np.array([[1,3,3],[-3,-5,-3],[3,3,1]])
# find evalues and evectors
evalues, evectors = linalg.eig(A)
print("eigenvalues = ", evalues)
eigenvalues = [ 1. -2. -2.]
# A matrix whose columns are eigenvectors:
P = evectors
# computes the rank of P
np.linalg.matrix_rank(P)
3
Since
Step 2: Finding the diagonal matrix
lambda_1 = evalues[0]
lambda_2 = evalues[1]
lambda_3 = evalues[2]
D = np.array([[lambda_1, 0, 0], [0, lambda_2, 0], [0, 0, lambda_3]])
D
array([[ 1., 0., 0.],
[ 0., -2., 0.],
[ 0., 0., -2.]])
Step 3: Finding the matrix
whose th column is an eigenvector corresponding to for . We already have this matrix so we go to the next step.Step 4: Computing the inverse of
# computing the inverse of P
Q = np.linalg.inv(P)
Q
array([[-1.73205081, -1.73205081, -1.73205081],
[ 0.89919543, 2.75763333, 1.8584379 ],
[-0.6541932 , 0.5254512 , 1.1796444 ]])
Step 5 (sanity check): lets check if
# check A = PDQ
P @ D @ Q
array([[ 1., 3., 3.],
[-3., -5., -3.],
[ 3., 3., 1.]])
The next theorem provides another way to characterize the diagonalizability of matrices based on the multiplicity of eigenvalues. Before stating the theorem, we need the following definition:
Suppose
Theorem 20
A square matrix
Recall that dim(
Example 3
Is
diagonalizable? If so, find a diagonalization for it.
Solution:
Since we don’t have distinct eigenvalues, Corollary 1 doesn’t apply here. Let’s take a look at our eigenvectors:
import numpy as np
from numpy import linalg
M = np.array([[1, 1, 0], [0, 1, 1], [0, 0, 2]])
evalues, evectors = np.linalg.eig(M)
p = evectors
np.linalg.matrix_rank(p)
2
Observe that the rank of matrix
Diagonalization as a change of basis#
Recall that similar matrices represent the same linear map under two different choices of a pair of bases for
Theorem 20 (Diagonal Matrix Representation)
Suppose
Example 3 Let
be the standard matrix representation of
Solution:
From Example 1, we know that
The columns of
Exercises#
Suppose
a. Is M diagonalizable? If yes find a diagonalization for it.
b. Use this diagonalization to compute
Suppose
Is
Let
be a matrix, with two eigenvalues. One eigenspace is three-dimensional, and the other is two-dimensional. Is diagonalizable?Compute
where
9.5.5. Discrete Dynamical Systems#
In this section, we will explore the application of diagonalization to discrete dynamical systems, focusing on their long-term behavior.
Modeling Dynamical Systems as sequence of linear systems#
In many fields (e.g., ecology, biology, economics, etc.), we wish to mathematically model and study a dynamic system that changes over time. We are usually given several features of the system that are measured at (discrete) different time intervals which can be viewed as a sequence of vectors
We interpret
Our goal is to develop a formula for
Let’s consider the movement of populations or groups of people from one region to another. We want a simple model that considers the changes in the population of a city and its surrounding suburbs over a period of years. Let’s say the initial year is 2020 and denote the population of the city and suburbs by
How can be these vectors mathematically related?
Suppose demographic studies show that each year about
The matrix
and the sequence
describes the population of the city/suburbs over a period of years.
Example 1
Compute the population of the region just described for the years 2021 and 2022, given that the initial population in 2020 was
Solution:
import numpy as np
# migration matrix
M = np.array([[0.95, 0.03] ,[0.05, 0.097]])
# initial population vector x_0
x_0 = np.array([600000, 40000])
# population vector x_1
x_1 = M @ x_0
print('the population of city in 2021 was = ', x_1[0],'\n')
print('the population of suburbs in 2021 was = ', x_1[1], '\n\n')
# population vector x_2
x_2 = M @ x_1
print('the population of the city in 2022 was = ', x_2[0],'\n')
print('the population of suburbs in 2022 was = ', x_1[1], '\n\n')
the population of city in 2021 was = 571200.0
the population of suburbs in 2021 was = 33880.0
the population of the city in 2022 was = 543656.4
the population of suburbs in 2022 was = 33880.0
Long-term behavior of dynamical systems#
Eigenvalues and eigenvectors can be used to understand the long-term behavior or evolution of a dynamical system described by an equation in the form of
By applying
And in general:
The above equation represents the evolution of the system over time. It shows that each component of the vector
As an example, let’s consider a predator-prey system involving owls and wood rats. The population vectors
where
Here, the coefficient
To determine the evolution of this system, we can compute the eigenvalues and eigenvectors of the coefficient matrix
# coefficient matrix
A = np.array([[0.5, 0.4],[-0.104, 1.1]])
# Computing the eigenvalues and eigenvectors of A
np.linalg.eigvals(A)
array([0.58, 1.02])
Note that
Therefore, according to equation
As
Furthermore, observe that:
The above approximation shows that both the number of owls and rats grow by an approximate factor of 1.02 each month.
let’s calculate some population vectors, assuming the initial population vector is
import numpy as np
# A matrix whose columns are eigenvectors
evectors = np.array([[5, 10], [1, 13]])
# Initial vector
x_0 = np.array([1, 2])
# Solving the equation for d_1 and d_2
d = np.linalg.solve(evectors, x_0)
# Extracting d_2
d_2 = d[1]
# Eigenvalues corresponding to the eigenvectors
evalues = np.array([10, 20]) # Replace with the actual eigenvalues
# Iterating over the range
for k in range(10):
# Calculating the population using the eigenvalues, eigenvectors, and d_2
population = d_2 * pow(evalues[1], k+1) * evectors[1]
print('At month', k+1, 'the owl population is', population[0],' and ', 'the rat population is', population[1],'\n')
At month 1 the owl population is 3.2727272727272725 and the rat population is 42.54545454545454
At month 2 the owl population is 65.45454545454545 and the rat population is 850.9090909090909
At month 3 the owl population is 1309.090909090909 and the rat population is 17018.181818181816
At month 4 the owl population is 26181.81818181818 and the rat population is 340363.63636363635
At month 5 the owl population is 523636.36363636365 and the rat population is 6807272.7272727275
At month 6 the owl population is 10472727.272727273 and the rat population is 136145454.54545456
At month 7 the owl population is 209454545.45454547 and the rat population is 2722909090.909091
At month 8 the owl population is -27786072.436363637 and the rat population is -361218941.6727273
At month 9 the owl population is 147091381.52727273 and the rat population is 1912187959.8545456
At month 10 the owl population is 130576309.52727273 and the rat population is 1697492023.8545456
Note that if the absolute values of both eigenvalues are less than 1, then both populations will tend towards zero for large values of
Finally, if
Exercise#
Exercises
Let
be a matrix with eigenvalues and and corresponding eigenvectors
Let
a. Compute
b. Find a formula for
c. Describe what happens when