import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
12.8. Solutions to Exercises#
12.8.1. Logistic Growth and COVID-19#
First, separate the variables and antidifferentiate both sides
The partial fraction decomposition
with
leads to
Considering the initial condition
2a)
Show code cell source
import matplotlib.pyplot as plt
import numpy as np
from scipy.integrate import solve_ivp
plt.style.use('seaborn-poster')
%matplotlib inline
k=1
y0a=10
y0b=50
y0c=90
y0d=110
M=100
F = lambda t,y: k*y*(1-y/M)
t_eval = np.arange(0, 10, 0.01)
sola = solve_ivp(F, [0, 10], [y0a], t_eval=t_eval)
solb = solve_ivp(F, [0, 10], [y0b], t_eval=t_eval)
solc = solve_ivp(F, [0, 10], [y0c], t_eval=t_eval)
sold = solve_ivp(F, [0, 10], [y0d], t_eval=t_eval)
plt.figure(figsize = (5,5))
plt.plot(sola.t, sola.y[0])
plt.plot(solb.t, solb.y[0])
plt.plot(solc.t, solc.y[0])
plt.plot(sold.t, sold.y[0])
plt.title('Logistic Growth')
plt.xlabel('t (in days)')
plt.ylabel('number infected')
plt.ylim((0,120))
plt.xlim((0,11))
plt.legend(['y0=10','y0=50','y0=90','y0=110'])
plt.show()
<ipython-input-1-b136c51ef48b>:5: MatplotlibDeprecationWarning: The seaborn styles shipped by Matplotlib are deprecated since 3.6, as they no longer correspond to the styles shipped by seaborn. However, they will remain available as 'seaborn-v0_8-<style>'. Alternatively, directly use the seaborn API instead.
plt.style.use('seaborn-poster')
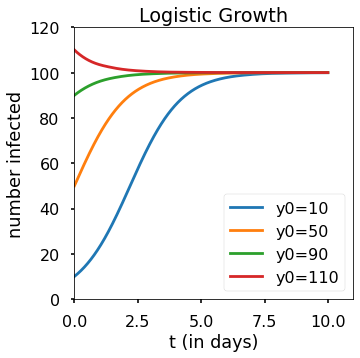
2b)
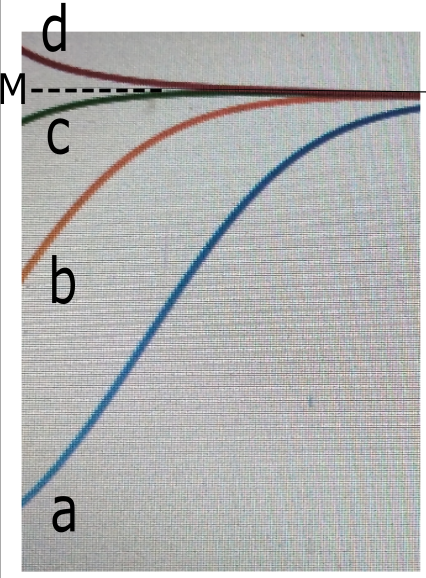
12.8.2. The Basic SIR Model#
Exercise 1
a) If the initial population is increased to 3,000,000, the epidemic appears to end sooner (around day 30)
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, R = y # unpack current values of y
beta, gamma # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [-beta * S * I,
beta * S * I - gamma * I,
gamma * I]
return derivs
# Parameters
beta=.000001
gamma=.2
# Initial values
S0=3000000
I0=1
R0=0
# Bundle parameters for ODE solver
params = [beta,gamma]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,R0]
# Make time array for solution
tStop = 70 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(t, psoln[:,0],label='susceptible')
plt.plot(t, psoln[:,1],label='infected')
plt.plot(t, psoln[:,2],label='recovered')
plt.legend()
plt.xlabel('day')
plt.ylabel('number')
plt.savefig("SIR.png")
plt.tight_layout()
plt.show()
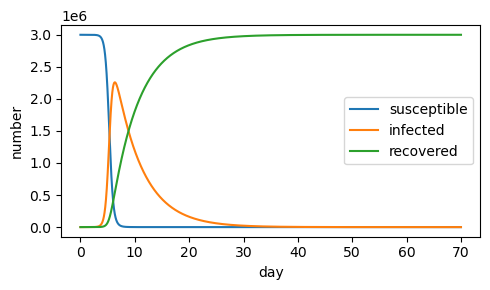
If the initial population is reduced to 1,000, there is no epidemic.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, R = y # unpack current values of y
beta, gamma # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [-beta * S * I,
beta * S * I - gamma * I,
gamma * I]
return derivs
# Parameters
beta=.000001
gamma=.2
# Initial values
S0=1000
I0=1
R0=0
# Bundle parameters for ODE solver
params = [beta,gamma]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,R0]
# Make time array for solution
tStop = 1000 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(t, psoln[:,0],label='susceptible')
plt.plot(t, psoln[:,1],label='infected')
plt.plot(t, psoln[:,2],label='recovered')
plt.legend()
plt.xlabel('day')
plt.ylabel('number')
plt.savefig("SIR.png")
plt.tight_layout()
plt.show()
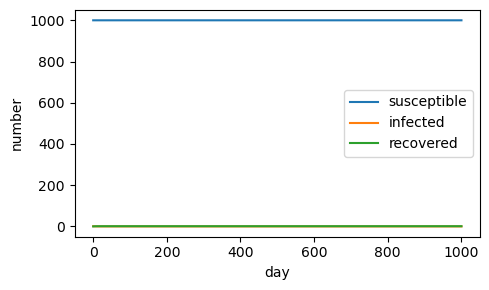
b) If the transmission rate is high (
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, R = y # unpack current values of y
beta, gamma # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [-beta * S * I,
beta * S * I - gamma * I,
gamma * I]
return derivs
# Parameters
beta=.0001
gamma=.2
# Initial values
S0=1000000
I0=1
R0=0
# Bundle parameters for ODE solver
params = [beta,gamma]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,R0]
# Make time array for solution
tStop = 70 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(t, psoln[:,0],label='susceptible')
plt.plot(t, psoln[:,1],label='infected')
plt.plot(t, psoln[:,2],label='recovered')
plt.legend()
plt.xlabel('day')
plt.ylabel('number')
plt.savefig("SIR.png")
plt.tight_layout()
plt.show()
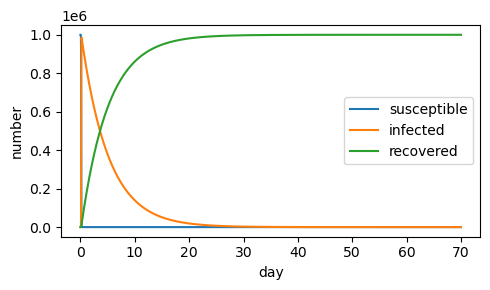
If the infection rate is reduced (
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, R = y # unpack current values of y
beta, gamma # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [-beta * S * I,
beta * S * I - gamma * I,
gamma * I]
return derivs
# Parameters
beta=.0000001
gamma=.2
# Initial values
S0=1000000
I0=1
R0=0
# Bundle parameters for ODE solver
params = [beta,gamma]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,R0]
# Make time array for solution
tStop = 70 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(t, psoln[:,0],label='susceptible')
plt.plot(t, psoln[:,1],label='infected')
plt.plot(t, psoln[:,2],label='recovered')
plt.legend()
plt.xlabel('day')
plt.ylabel('number')
plt.savefig("SIR.png")
plt.tight_layout()
plt.show()
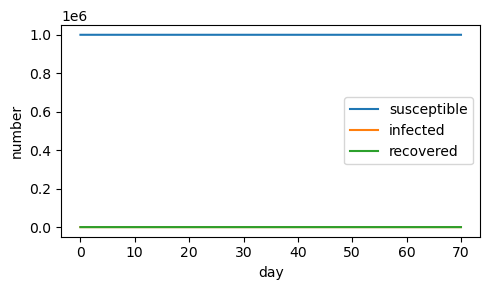
c) If the recovery rate is increased (
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, R = y # unpack current values of y
beta, gamma # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [-beta * S * I,
beta * S * I - gamma * I,
gamma * I]
return derivs
# Parameters
beta=.000001
gamma=.3
# Initial values
S0=1000000
I0=1
R0=0
# Bundle parameters for ODE solver
params = [beta,gamma]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,R0]
# Make time array for solution
tStop = 70 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(t, psoln[:,0],label='susceptible')
plt.plot(t, psoln[:,1],label='infected')
plt.plot(t, psoln[:,2],label='recovered')
plt.legend()
plt.xlabel('day')
plt.ylabel('number')
plt.savefig("SIR.png")
plt.tight_layout()
plt.show()
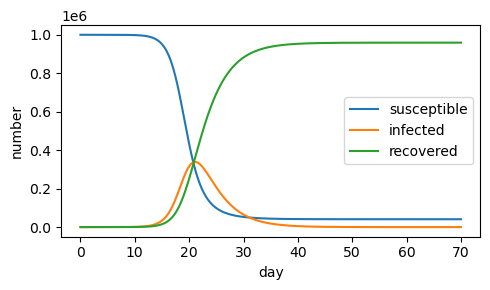
If the recovery rate is decreased (
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, R = y # unpack current values of y
beta, gamma # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [-beta * S * I,
beta * S * I - gamma * I,
gamma * I]
return derivs
# Parameters
beta=.000001
gamma=.1
# Initial values
S0=1000000
I0=1
R0=0
# Bundle parameters for ODE solver
params = [beta,gamma]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,R0]
# Make time array for solution
tStop = 70 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(t, psoln[:,0],label='susceptible')
plt.plot(t, psoln[:,1],label='infected')
plt.plot(t, psoln[:,2],label='recovered')
plt.legend()
plt.xlabel('day')
plt.ylabel('number')
plt.savefig("SIR.png")
plt.tight_layout()
plt.show()
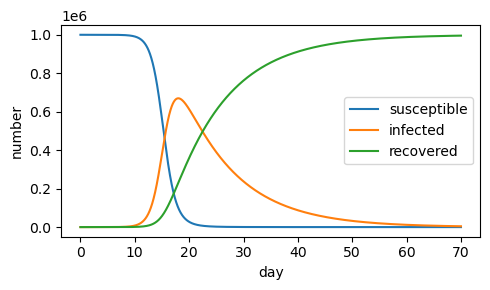
d) As we saw in part b), with a high enough transmission rate, the entire population can become infected.
e) As we also saw in part b), with a low enough transmission rate, there is no outbreak.
Exercise 2
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def SIRV(y, t, params):
S, I, R, V = y # unpack current values of y
beta, gamma, v # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [-beta * S * I - v*V,
beta * S * I - gamma * I,
gamma * I,
v*S]
return derivs
# Parameters
beta=.000001
gamma=.2
v=.01
# Initial values
S0=3000000
I0=1
R0=0
V0=0
# Bundle parameters for ODE solver
params = [beta,gamma,v]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,R0,V0]
# Make time array for solution
tStop = 70 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(SIRV, y0, t, args=(params,))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(t, psoln[:,0],label='susceptible')
plt.plot(t, psoln[:,1],label='infected')
plt.plot(t, psoln[:,2],label='recovered')
plt.plot(t, psoln[:,3],label='vaccinateded')
plt.legend()
plt.xlabel('day')
plt.ylabel('number')
plt.savefig("SIRV.png")
plt.tight_layout()
plt.show()
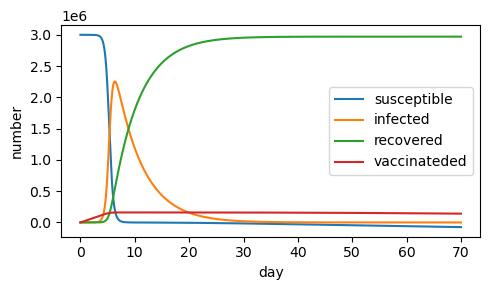
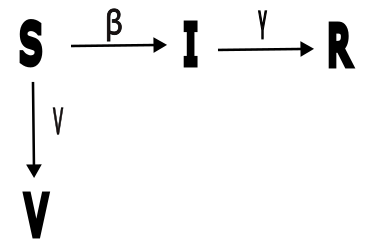
Exercise 3
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def SIRD(y, t, params):
S, I, R, D= y # unpack current values of y
beta, gamma, mu # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [-beta * S * I,
beta * S * I - gamma * I - mu*I,
gamma * I,
mu*I]
return derivs
# Parameters
beta=.000001
gamma=.2
mu=.01
# Initial values
S0=3000000
I0=1
R0=0
D0=0
# Bundle parameters for ODE solver
params = [beta,gamma,mu]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,R0,D0]
# Make time array for solution
tStop = 70 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(SIRD, y0, t, args=(params,))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(t, psoln[:,0],label='susceptible')
plt.plot(t, psoln[:,1],label='infected')
plt.plot(t, psoln[:,2],label='recovered')
plt.plot(t, psoln[:,3],label='deceased')
plt.legend()
plt.xlabel('day')
plt.ylabel('number')
plt.savefig("SIRD.png")
plt.tight_layout()
plt.show()
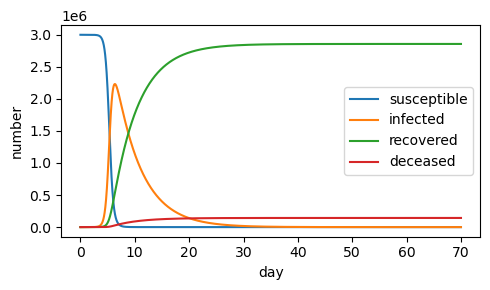
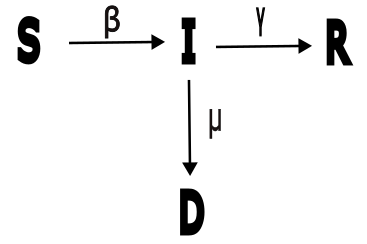
12.8.3. Cholera in Haiti#
Exercise 1
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, B = y # unpack current values of y
H,n,a,K,r,Nb,e # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [n*(H-S)-a*B*S/(K+B),
a*B*S/(K+B)-r*I,
B*Nb+e*I]
return derivs
# Parameters
H=10000
n=.0001
K=10**6
r=.2
Nb=-.33
e=10
# Initial values
S0=10000
I0=100
B0=0
exposure_rate=[]
max_infected=[]
for a in np.arange(0,100,1):
exposure_rate.append(a)
# Bundle parameters for ODE solver
params = [H,n,a,K,r,Nb,e]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,B0]
# Make time array for solution
tStop = 10 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
max_infected.append(np.max(psoln[:,1]))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(exposure_rate, max_infected,label='max infected')
plt.legend()
plt.xlabel('a=exposure rate')
plt.ylabel('max infected population')
plt.savefig("cholerEx1.png")
plt.tight_layout()
plt.show()
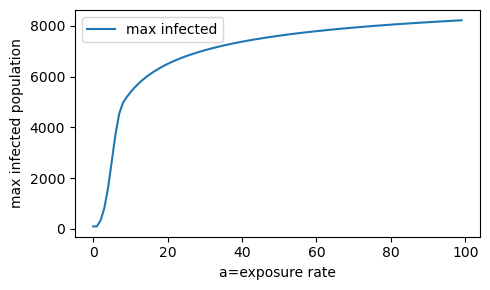
The max infected population rises sharply as the exposure rate (a) is increased from 0 to 10. When a=100, the max_infected population has leveled off at approximately 8000 people.
Exercise 2
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, B = y # unpack current values of y
H,n,a,K,r,Nb,e # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [n*(H-S)-a*B*S/(K+B),
a*B*S/(K+B)-r*I,
B*Nb+e*I]
return derivs
# Parameters
H=10000
n=.0001
K=10**6
r=.2
Nb=-.33
# Initial values
S0=10000
I0=100
B0=0
contam=[]
peak1=[]
peak2=[]
for e in np.arange(0,50,1):
contam.append(e)
a=40
# Bundle parameters for ODE solver
params = [H,n,a,K,r,Nb,e]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,B0]
# Make time array for solution
tStop = 10 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
peak1.append(np.max(psoln[:,1]))
a=20
# Bundle parameters for ODE solver
params = [H,n,a,K,r,Nb,e]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,B0]
# Make time array for solution
tStop = 10 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
peak2.append(np.max(psoln[:,1]))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(contam, peak1,label='max infected (a=40)')
plt.plot(contam, peak2,label='max infected (a=20)')
plt.legend(["a=40","a=20"])
plt.xlabel('e=water contamination rate')
plt.ylabel('max infected population')
plt.savefig("cholerEx2.png")
plt.tight_layout()
plt.show()
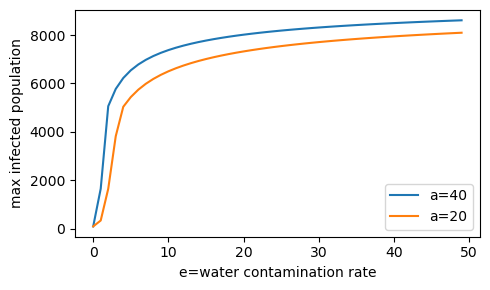
Increased exposure rate (a=20 to a=40) increases the max infected population at each level of the water contamination rate (e).
Exercise 3
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, B = y # unpack current values of y
H,n,a,K,r,Nb,e # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [n*(H-S)-a*B*S/(K+B),
a*B*S/(K+B)-r*I,
B*Nb+e*I]
return derivs
# Parameters
H=10000
n=.0001
K=10**6
e=10
Nb=-.33
# Initial values
S0=10000
I0=100
B0=0
recovery=[]
peak1=[]
peak2=[]
for r in np.arange(0,.8,.01):
recovery.append(r)
a=40
# Bundle parameters for ODE solver
params = [H,n,a,K,r,Nb,e]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,B0]
# Make time array for solution
tStop = 10 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
peak1.append(np.max(psoln[:,1]))
a=80
# Bundle parameters for ODE solver
params = [H,n,a,K,r,Nb,e]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,B0]
# Make time array for solution
tStop = 10 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
peak2.append(np.max(psoln[:,1]))
# Plot results
fig = plt.figure(1, figsize=(5,3))
plt.plot(recovery, peak1,label='max infected (a=40)')
plt.plot(recovery, peak2,label='max infected (a=80)')
plt.xlabel('r=recovery rate')
plt.ylabel('max infected population')
plt.savefig("cholerEx3.png")
plt.tight_layout()
plt.show()
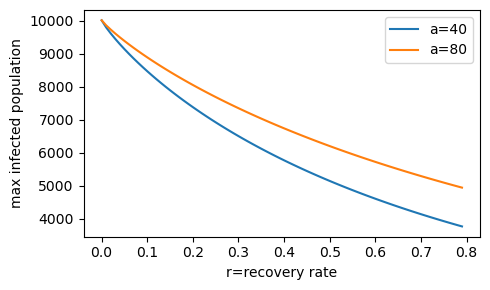
Peak infection dependency on the cholera recovery rate r for two exposure levels (a=40/day and a=80/day).
Exercise 4
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define Equations
def f(y, t, params):
S, I, B = y # unpack current values of y
H,n,a,K,r,Nb,e # unpack parameters
#specify dS/dt, dI/dt and dR/dt
derivs = [n*(H-S)-a*B*S/(K+B),
a*B*S/(K+B)-r*I,
B*Nb+e*I]
return derivs
# Parameters
H=10000
n=.0001
K=10**6
r=.2
e=10
Nb=-.33
# Initial values
S0=10000
I0=0
Z=np.eye(101, 201)
for B0 in np.arange(0,100,1):
for i in np.arange(0,200,1):
a=i/10 # exposure rate
# Bundle parameters for ODE solver
params = [H,n,a,K,r,Nb,e]
# Bundle initial conditions for ODE solver
y0 = [S0,I0,B0]
# Make time array for solution
tStop = 10 # number of days
tInc = .001
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
# Record the peak infection
Z[B0,i]=np.max(psoln[:,1])
# Plot results
# Creating dataset
x=np.arange(0,100,1)
y=np.arange(0,200,1)
# Creating figure
fig = plt.figure()
# syntax for 3-D projection
ax = plt.axes(projection ='3d')
for i in np.arange(1,100,1):
for j in np.arange(0,200,1):
ax.scatter(i, j, Z[i,j],color='lightgray')
ax.set_title('Max Infected')
ax.set_xlabel('a= .1 * i = exposure rate')
ax.set_ylabel('B0=initial bacteria concent')
ax.set_zlabel('Max Infected')
plt.savefig("cholerEx4.png")
plt.show()
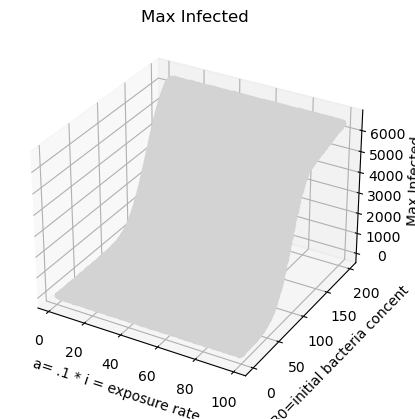
Exercise 5
a) Though it would be better to revise the model by adding a vaccinated compartment V (t), the basic model might reflect a vaccination program by lowering the initial susceptible population S(0) and raising the value of K, the latter being the bacteria concentration required to give a .5 probability.
b) Seasonal behavior of cholera outbreaks which may be related to weather events such as floods or El Nino must be considered. That is, even though there may be a period with no or very few cases, there is a latent possibility for re-emergence of a large number of infected population. Mathematically viable solutions may be overly simplistic in light of the reality of everyday life in Haiti.
From the sensitivity analysis, the contamination rate seems to have the largest effect on the infected population, although exposure rate, recovery rate, and initial concentration of bacteria also have an effect. Within the range of 2.5-30 number of people contaminated per day, the infected population will increase significantly. As the contamination rate increases above 30, the infected population stabilizes and increases more slowly. These observations suggest focusing response and preparation measures on lowering the contamination rate
12.8.4. CWS Model of Alzheimer’s Disease#
Exercise 1
Exercise 2
The total exit rate of monomers from the CSF (
Exercises 3
Treatment 2 shows that the plasma and CSF levels of amyloid-beta monomers might increase, but the level in the brain actually decreases.
Exercise 4
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
def f(y, t, params):
b1, c,p = y # unpack current values of y
k1,k2,k3, r12,r13,r23,r21,r31,r32,L1,L2,L3,E,F,S = params # unpack parameters
derivs = [k1+F*S+r31*p+r21*c-(r13*b1+r12*b1*(1+np.heaviside(t-100,0)-np.heaviside(t-465,0)))-(L1+E*S)*b1-2*E*b1**2, # list of dy/dt=f functions
k2+r12*b1*(1+np.heaviside(t-100,0)-np.heaviside(t-465,0))+r32*p-(r21*c+r23*c)-L2*c,
k3+r13*b1+r23*c-(r31*p+r32*p)-L3*p]
return derivs
# Parameters
k1 = 7.34*86400
L1 = 24
k2=0
L2=0
k3=0
L3=6.7*10**(-3)*86400
r12=7.6*10**(-6)*86400
r23=1.7*10**(-3)*86400
r31=3.7*10**(-5)*86400
r32=0
r21=0
r13=0
E=0
F=.238
S=0
# Initial values
b10 = 25.7*(10**3)
c0 = 115
p0 = 29
# Bundle parameters for ODE solver
params = [k1,k2,k3, r12,r13,r23,r21,r31,r32,L1,L2,L3,E,F,S]
# Bundle initial conditions for ODE solver
y0 = [b10,c0,p0]
# Make time array for solution
tStop = 1000
tInc = 0.05
t = np.arange(0., tStop, tInc)
# Call the ODE solver
psoln = odeint(f, y0, t, args=(params,))
# Plot results
fig = plt.figure(1, figsize=(8,8))
# Plot b1 as a function of time
ax1 = fig.add_subplot(311)
ax1.plot(t, psoln[:,0])
ax1.set_xlabel('time')
ax1.set_ylabel('b1')
# Plot c as a function of time
ax2 = fig.add_subplot(312)
ax2.plot(t, psoln[:,1])
ax2.set_xlabel('time')
ax2.set_ylabel('c')
# Plot p as a function of time
ax3 = fig.add_subplot(313)
ax3.plot(t, psoln[:,2])
ax3.set_xlabel('time')
ax3.set_ylabel('p')
plt.savefig("treatment2nonlin.png")
plt.tight_layout()
plt.show()
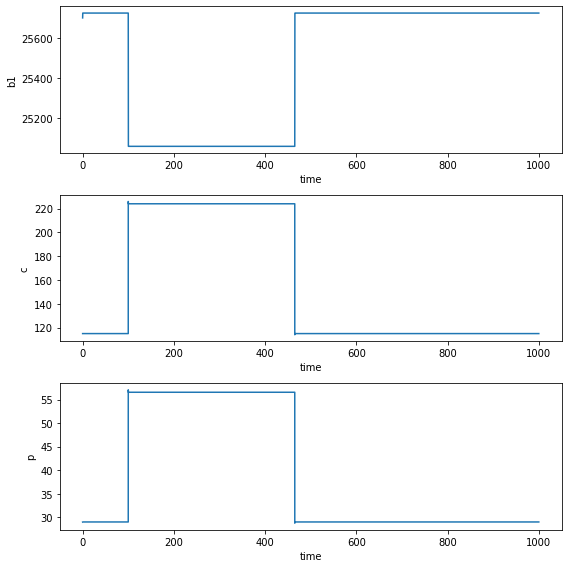
The output of the nonlinear is the same as the simplified linear model since the former is equivalent to the latter when
12.8.5. Gravity-Fed Water Delivery#
[2.1]
[2.2]
[2.3] Since
[3.1.1]
[a] Bernoulli’s equation states
[b] From part a we know
[3.1.2] Referring to Figure (S1), let
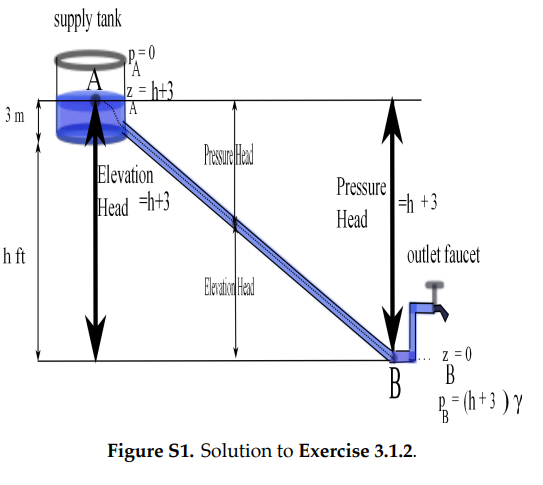
[3.2.1] [a] At a horizontal distance of 600 m, pressure is greatest since the vertical drop from the source is a maximum (114 m).
[b] The pipe can operate at a pressure head of
[3.2.2] Pressure at A is equal to
[3.3.1] .1215 liters/sec.
[3.3.2]
[a] A velocity head of 3 m (10 ft) corresponds to a speed
[b]
[4.1]
A negative pressure means that pressure inside the pipe is less than outside. This can cause dirt or air to be sucked into the pipe, resulting in a stoppage of flow.
[4.2] The Reynolds number
[4.3.1]
[4.3.2]
[4.3.3] Since the pipe is wider at B than at A,
[4.3.4]
$
The flow rate would be
So for a very reasonable
[4.4.1] The iron pipe has the greater friction factor.
[4.4.2]
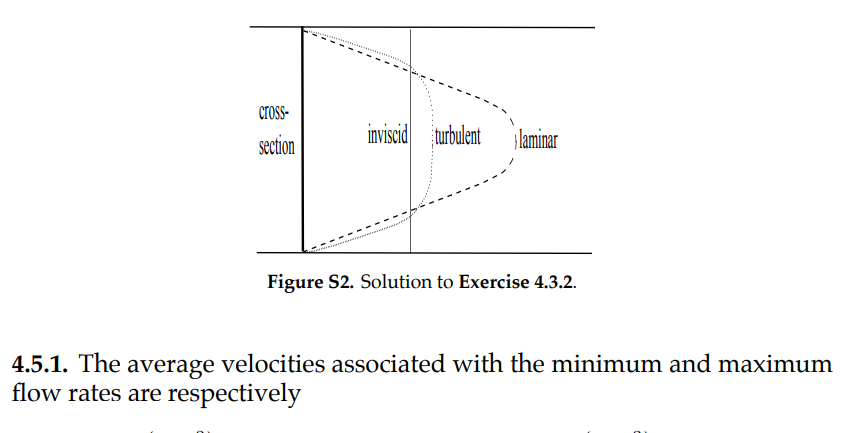
[4.5.1] The average velocities associated with the minimum and maximum flow rates are respectively
[4.5.2] Using the equation for
and
Knowing the head loss, we can easily plot the HGL on the graph shown in Figure (21). The HGL will help us decide whether the pipe may be used for that segment of the system as explained in Section 4.4.
[5.1] There are different possible designs, but a system using a 80 mm diameter is shown here. In 10 years Tesuque Pueblo will have about 1148 people and a required volumetric flow rate of
12.8.6. Earthquake Resistant Construction#
2.1
a)
b)
c)
d)
2.2 For example, to check the solution for
It follows easily that
2.3 For
and
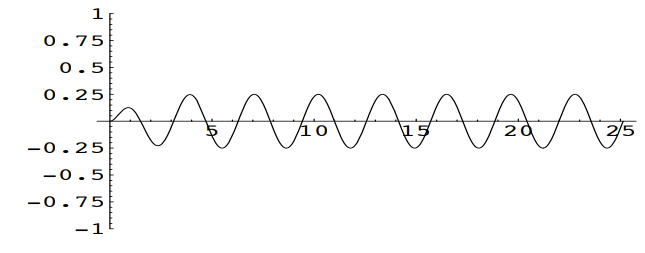
At the critical value
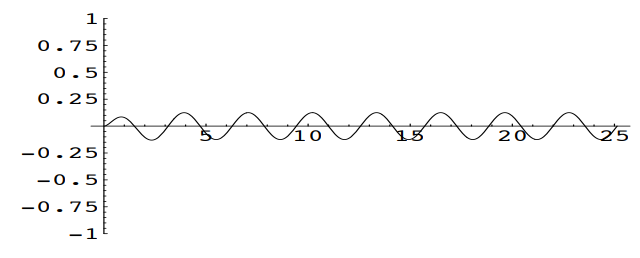
Note that in general, increasing the damping coefficient
2.4 Since
2.4.1
2.4.2 a)
b)
$
c)
2.5
The ground motion
3.1
3.3
3.4