8.7. Sequences and Series#
Tip
Below is the list of topics that are covered in this section:
Sequences
Series
Convergence and Divergence of Series
Convergence Tests
Power Series
import matplotlib.pyplot as plt
import math
8.7.1. Sequences#
In this module, we define a sequence as an arrangement of an infinite number of numbers written in a specific order.
The common notations used for a sequence are
Example
Write the first 5 terms of the following sequences:
Some common sequences:
Arithmetic sequence: created by adding a certain constant
to the preceding term.Using
, the sequence is written explicitly:Or, written recursively:
where and for .
Geometric sequence: created by multiplying a certain constant ratio
to the preceding term.Using
, the sequence is written explicitly:Or, written recursively:
where and for .
Harmonic sequence: created by taking the reciprocal of an arithmetic sequence.
Using
, the sequence is written explicitly:
Fibonacci sequence: created by taking each term as the sum of the two preceding terms.
Written recursively:
where , and for
Properties of Sequences#
Given a sequence
is increasing if for every , also called monotonic increasing. is decreasing if for every , also called monotonic decreasing. is monotonic nonincreasing (or monotonic nondecreasing) if never increase (or decrease). is bounded below if there exist a number such that for every . Here is called a lower bound. is bounded above if there exist a number such that for every . Here is called a upper bound. is bounded if it is both bounded below and bounded above.
Example
Determine if the given sequence is monotonic and/or bounded.
is monotonic (decreasing) as for every . is bounded since for every . The first term is , value kept decreasing, but no matter how big is, is never negative.
is NOT monotonic since the sequence terms alternate between and so neither an increasing nor a decreasing sequence. is bounded since for every .Note that the lower bound and upper bound are not unique. We can also said
is bounded since for every .
Below is an example code that plot the first 100 terms of the sequence
Show code cell source
# Ask the user for the sequence
sequence = input("Enter the sequence {a_n}: ")
# Evaluate the sequence for the first 100 terms (can change the range as needed)
terms = []
for n in range(1, 101):
term = eval(sequence, {'n': n})
terms.append(term)
# Create the plot with dots
plt.scatter(range(1, 101), terms)
plt.xlabel("n")
plt.ylabel("a_n")
plt.title("Plot of the Sequence {a_n}")
plt.grid(True)
# Display the plot
plt.show()
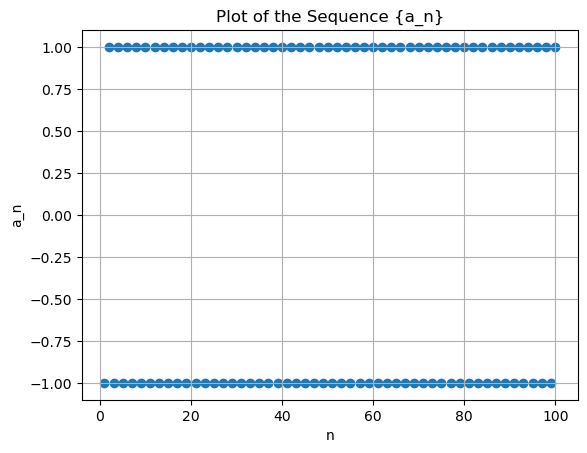
Limit of Sequences#
Recall limit notation from the Limit Section.
We say that
if for every number there is an integer such that whenever .We say that
if for every number there is an integer such that whenever .We say that
if for every number there is an integer such that whenever .
If
If
Note that these limit definitions are not the same with monotonicity, which is stricter.
The use of an integer
The sequence
(the rest of the terms are all ).> is not monotonic, but since at some point, all the term goes to .
The sequence
(the rest of the terms keep going down by ). is not monotonic decreasing, but since at some point the term kept going down.
Properties of limits of sequences follow from the properties of limits, so will not be relisted in this section.
Theorems#
Below are some useful theorems on sequences. Motivated students are encouraged to prove them.
Theorems
Theorem 1: If
Theorem 2: If
Theorem 3: The sequence
Theorem (Squeeze Theorem for Sequences): If
While the theorems are useful, it is also useful to be able to check the convergence or divergence of a sequence using Python code.
Below is a code that checked the first 100 terms of
Show code cell source
# Ask the user for the sequence
sequence = input("Enter the sequence a_n: ")
# Evaluate the first 100 terms of the sequence
terms = []
for n in range(1, 101):
term = eval(sequence, {'n': n, 'math': math})
terms.append(term)
# Check if the sequence converges or diverges
converges = all(term == terms[0] for term in terms)
if converges:
print("The sequence converges.")
else:
print("The sequence diverges.")
The sequence diverges.
8.7.2. Series#
(Infinite) series are an infinite sum of terms
Example
The
What letter used to represent the index does not matter:
, etc.For convenience, index shift can be applied to series:
, etc.With this in mind, it is normal for different resources and math literatures to define series with index started with
.
Example
8.7.3. Convergence and Divergence of Series#
The
The series
The series
Example
is convergent since . is divergent since .
Properties of Convergent Series#
If
is also convergent for any constant . is also convergent.
Recall distributive property to note that
No conclusions can be drawn about the convergence or multiplication of series. Moreover, given how complicated the multiplication of infinite series is, people would rather avoid doing it.
Theorems
Theorem: If
Theorem (Divergence Test): If
Example
The series
Geometric Series#
A geometric series is a series that can be written in the form
The
Taking the limit of the sequence of partial sums:
The only term depending on
Example
Determine if
The convergence of this series can be seen by rewriting it in geometric series form and calculating:
Harmonic Series#
A harmonic series is the series
8.7.4. Convergence Tests#
Integral Test#
If
if
is convergent then is also convergent.if
is divergent then is also divergent.
Example: Harmonic Series
Note that
Since
The -series Test#
If
Example
Comparison Test#
Given two series
If
is convergent then is also convergentIf
is divergent then is also divergent
Example
is convergent, because and is convergent as it is a -series with . is divergent, because and is divergent as it is a -series with .
Limit Comparison Test#
Given two series
If
Limit Comparison Test (LCT) is similar to the Comparison Test (CT) in the sense that we want to compare the series with a series that we know or easily recognize as converging or diverging.
Example
Is
Take
As
Alternating Series Test#
Suppose
If
Example
Determine if
Note that
Since
Absolute and Conditional Convergence#
The series
The series
It immediately follows that absolute convergence implies convergence, but not vice versa.
Example
is convergent by the Alternating Series Test since it’s a Harmonic series (by the Integral Test).
Ratio Test#
Given the series
If
then the series is absolutely convergent, and hence convergentIf
then the series is divergentIf
then the test is inconclusive
Example
is absolutely convergent since
is divergent since .Ratio Test fails for determining convergence of
since .However, we knew from
-test that is convergent.
Root Test#
Given the series
If
then the series is absolutely convergent, and hence convergentIf
then the series is divergentIf
then the series is inconclusive
In using the Root Test, we often use the fact that
Example
is absolutely convergent since . is divergent since .The Root Test fails for determining convergence of
since .However, we already know that this Harmonic series is divergent.
Convergence Test with Python#
While multiple tests can be used to manually determine the convergence of a series with steps that can become complicated, in Python, testing the convergence of a series is built in.
Below is a code that ask for
Show code cell source
# Ask the user for the sequence
sequence = input("Enter the sequence a_n: ")
# Define the symbol n
n = sp.Symbol('n')
# Define the series sum(a_n) from n=1 to infinity
series = sp.Sum(eval(sequence), (n, 1, sp.oo))
# Check if the series is convergent or divergent
if series.is_convergent():
print("The series is convergent.")
else:
print("The series is divergent.")
The series is convergent.
8.7.5. Power Series#
A power series (about
Since power series are a function of
The radius of convergence of a power series is the number
The interval of convergence is the interval containing all values of
Example
Determine the radius and interval of convergence for
Using Ratio Test:
By Ratio Test,
Hence the radius of convergence is 0.5.
For the interval of convergence, solving for
at
, the sum is , which converges by the Alternating Series Test.at
, the sum is , which diverges since it is a Harmonic series.
Thus, the interval of convergence is
8.7.6. Exercises#
Exercises
List the first 5 terms of each given sequence, then determine whether each sequence is monotonic and/or bounded.
Determine whether the following sequences converge or diverge. Determine the limit if it exists.
Index shift and rename exercise:
Write
as a series that starts at .Write
as a series that starts at .
Determine the convergence of the following series:
Determine the interval and radius of convergence of the following series: