5.2. Random Numbers#
Many areas of study involve random numbers. Examples include probability, computational science, data science, and statistics.
5.2.1. Arbitrariness#
When you tell a friend to “Pick a number between 1 and 10,” you may think you are telling the friend to pick a random number. However, for true randomness, each outcome 1, 2, 3, …, 10 must be equally likely. It turns out that people have a preference, usually unconscious, for picking some numbers over others. Therefore, you are really telling the friend to pick an arbitrary integer between 1 and 10, rather than a random one.
5.2.2. Pseudo-randomness#
Even when computers do the choosing, most of time we should only say we have generated pseudo-random (fake random) numbers. A pseudo-random number generator, or PRNG, is a program that uses mathematical methods to simulate (mimic) randomness.
The basic process is to:
Provide the PRNG with an arbitrary seed (starting condition).
Ask the PRNG for the next “random” number. It is produced by a step-by-step, complicated, but not random, process.
Such a generator is also called a deterministic random bit generator. “Deterministic” means causally determined by preceding actions. The word “deterministic” is typically used in contrast to “random.”
Sometimes, researchers use a cryptographically secure pseudo-random number generator (CSPRNG). A CSPRNG may sound like it uses true randomness (for strong security). However, you cannot safely assume that you have a completely fair (unbiased) “coin flip” or means of selection, even in this context. Fortunately, though, the prescriptive (deterministic) mechanism involved is hard to reverse, ensuring some measure of security. The key is to have a CSPRNG that is good enough for the application at hand.
5.2.3. Pseudo-random number generation in Python#
People typically speak of computers generating random numbers. We will use this language when we use Python. However, Python (or Excel, Java, C, C++, or R) is technically doing pseudo-random number generation.
In Python, a default mechanism is used to set the seed for the PRNG. If you want to use the default mechanism explicitly, you can import therandom
library and do
import random
random.seed()
If for any reason you want to set the seed to a specific integer, such as 123, you can do
random.seed(123)
5.2.4. True randomness#
A truly random number cannot simply be generated from a certain algorithm (detailed method) or by a computer itself (unless the computer is purposely re-designed). Truly-random number generation is possible when we consider some physical phenomenon or process, such as atmospheric noise (static from lightning discharges), cosmic background radiation, etc. While truly random numbers are useful in many practical fields, it is sufficient for us to generate and use pseudo-random numbers in this book.
5.2.5. Exercises#
Exercises
Exercise 1. Which statement do you judge to be more accurate? Why?
The computer program selected a number between 1 and 10 at random.
The computer program selected a number between 1 and 10 arbitrarily.
Exercise 2. When a computer program selects a number between 1 and 10, do you think that the choice involves possible preference for some number over others? Support your answer with examples.
Exercise 3: Think of an example of how randomness might be involved in a study investigating a new medicine. Explain how randomness is involved. Could computation, for example using Python, be helpful in the study? Explain your answer.
Exercise 4: Think of an example of how randomness might occur in the natural world. Explain how randomness is involved. Could a computer simulate or mimic a natural process in the context of your example? If so, how could a computer simulation be useful?
Exercise 5: Think of an example of how randomness might occur in a game. Explain how randomness is involved. Could a computer simulate the game? If so, how could the computer simulation be useful?
Exercise 6: Consider a die (plural: “dice”). (See the image below.) Do you think that when we roll a physical die, we can be certain that there is an equal chance of the die landing with each of the six sides facing up? Or could there be a bias toward some sides? Explain your thinking. Do you think the outcome of a roll of the physical die could be influenced by the previous roll? Explain your thinking. How could we use a computer program to simulate a die roll? What are some advantages of doing so?
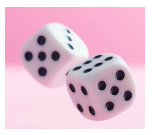
Exercise 7: Consider the following answer to the question “True or false— An arbitrary choice is made for no special reason”:
“Yes, an arbitrary choice typically refers to a decision or selection that is made without any specific reason or justification. It means that there is no objective or rational basis for choosing one option over another. Arbitrary choices are often made when there is no clear preference or when the decision doesn’t have a significant impact on the outcome. In such cases, people may resort to random selection, personal whim, or other non-logical factors to make a choice.” (ChatGPT, personal communication, June 6, 2023).
Provide a critique for which parts are right (if any). Provide justification for why they are correct. Identify which parts are wrong (if any) and explain what is wrong. Rewrite the answer in your own words with any corrections that are needed. (Retain the citation as with any paraphrased material.) Add an example that you think of yourself to clarify the answer to the true/false question.
Exercise 8: Use the code below to explore Python commands in therandom
library. Read the documentation here as desired: https://docs.python.org/3/library/random.html . For eachprint
statement, insert a comment (using#
) that describes all the possible numbers that can be generated. We see possible output below.
Exercise 9: Which does a Python program generate: a pseudo-random number or a truly random number? Give an example, and explain why the example is pseudo random or truly random.
print(random.randrange(6))
print(random.randrange(6) + 1)
print(random.randrange(1, 7))
print(random.randint(1, 6))
print(random.randrange(0, 10, 2))
print(random.uniform(10, 20))
0
3
1
4
4
11.07700234938439